Sequences and Summations
Contents
Sequences and Summations#
Objectives
Generate terms of a binomial sequence
Form sequences of partial sums
Look for patterns in partial sums
Problem: Sennett#
An ancient egyptian game of sennet involves dropping two colored sticks and moving a board piece based on the resulting stick color combination. Your goal is to complete the table below, and determine the number of ways for each outcome for 3, 4, and 5 sticks to occur.
num sticks |
0 red |
1 red |
2 red |
3 red |
4 red |
5 red |
---|---|---|---|---|---|---|
1 |
1 |
1 |
– |
– |
– |
– |
2 |
1 |
2 |
1 |
– |
– |
– |
3 |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
4 |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
5 |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
\(~\) |
Problem: Partial Sums#
Given the sequence below, we aim to evaluate the sum of a sequence and form a sequence based on these sums. For example, take the sequence:
the sequence formed by the sum of the first \(n\) terms would be:
Your goal for each of the following sequences is to create a sequence of partial sums, and try to generate a recursive and closed form definition of that sequence of sums.
\(a_n = 1, 2, 3, 4, 5, ...\)
\(a_n = 1^3, 2^3, 3^3, 4^3\)
The Big Idea and Notation#
Create a sequence, and create a sequence from sums of the terms of the sequence. Let’s move to a slightly different expression of these ideas where we form sequences based on functions defined in closed form. For each of the examples below use:
to evaluate the given function \(f\). Create a spreadsheet and visualize \(f(x)\). Next, create a sequence of partial sums on the same sheet and plot the sequence of partial sums alongside the functions.
\(f(x) = 1\)
\(f(x) = x\)
\(f(x) = x^2\)
\(f(x) = x^3\)
\(f(x) = x^4\)
Using Python and Notebooks#
While google sheets are nice, and there are many spaces that you may use a spreadsheet – we want to move to some more powerful and flexible tools. To begin, we aim to use Python to perform the operations we have used to this point. These are:
Define and use functions
Visualize functions
Form sequences of partial sums in Python
Some Basics#
Addition:
+
Subtraction:
-
Multiplication:
*
Division:
/
Exponents:
**
#addition
3 + 4
7
#subtraction
3 - 8
-5
#multiplication
2*10
20
#division
10/3
3.3333333333333335
#exponents
2**4
16
Defining functions#
def f(x):
return x**2
The above code defines a function that will take in a number and return its square. After running a code cell with this definition you can use the function with f(2)
.
#define a function
def f(x):
return x**2
#use the function
f(2)
4
Use the functions defined below to evaluate the following:
\(f(0)\)
\(g(0)\)
\(f(10)\)
\(g(.10)\)
\(g(0)\)
def f(x):
return x - 4
def g(x):
return 1/x
#f(0)
#g(0)
#f(10)
#g(.1)
#g(0)
Libraries and Plotting#
To create domains for our functions, we will use a library called numpy
. To visualize the functions, we will use a library called matplotlib
. In order to make use of these we first import and alias them below.
import matplotlib.pyplot as plt
import numpy as np
First, we will use numpy to create a domain as we did earlier with
x = np.arange(1, 11)
np.arange(1, 11)
array([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
np.arange(1, 11, .5)
array([ 1. , 1.5, 2. , 2.5, 3. , 3.5, 4. , 4.5, 5. , 5.5, 6. ,
6.5, 7. , 7.5, 8. , 8.5, 9. , 9.5, 10. , 10.5])
np.arange(0, 1, .1)
array([0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9])
#define the function
def f(x):
return x**2
#define the domain
x = np.arange(1, 11)
#evaluate function on domain
f(x)
array([ 1, 4, 9, 16, 25, 36, 49, 64, 81, 100])
Problems#
For each of the following, use python and numpy to define and evaluate the functions on the given domain.
\(f(x) = x^2 + 3x + 1\) where \(x1 = [0, 1, 2, 3, 4]\)
\(g(x) = 1/x\) where \(x2 = [1, 2, 3, 4, 5, 6, 7, 8]\)
\(h(x) = x^3\) where \(x3 = [0, .1, .2, ..., 1.9, 2.0]\)
#define f
#define x1
#define g
#define x2
#define h
#define x3
Plotting Functions with matplotlib
#
To plot a given function, use plt.plot(x, y)
. This produces a basic plot of a function or sequence. Below, the functions and domains from the previous three problems will be used to create plots below.
#plt.plot(x1, f(x1))
#plt.plot(x2, g(x2))
#plt.plot(x3, h(x3))
Computing Parital Sums#
To compute the partial sums of a sequence, use the np.cumsum
function. This computes the cumulative sum of a given sequence.
def f(x): return x
x = np.arange(1, 11)
np.cumsum(x)
array([ 1, 3, 6, 10, 15, 21, 28, 36, 45, 55])
plt.plot(x, np.cumsum(f(x)), '-o')
[<matplotlib.lines.Line2D at 0x7fea5d7abb20>]
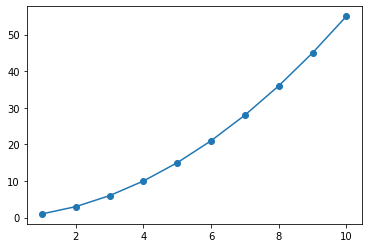
Summary#
Today, we began using partial sums to form new sequences from old. You want to get practice defining and plotting functions in python as we had above. Below are additional examples to use as practice with defining and plotting functions.
\(f(x) = \frac{x-2}{3x + 8}\)
\(f(x) = 9\)
\(f(x) = (x - 4)^2 + 5\)
\(f(x) = \sqrt{x^2 - 3x}\)